How To Download Video From Youtube Python
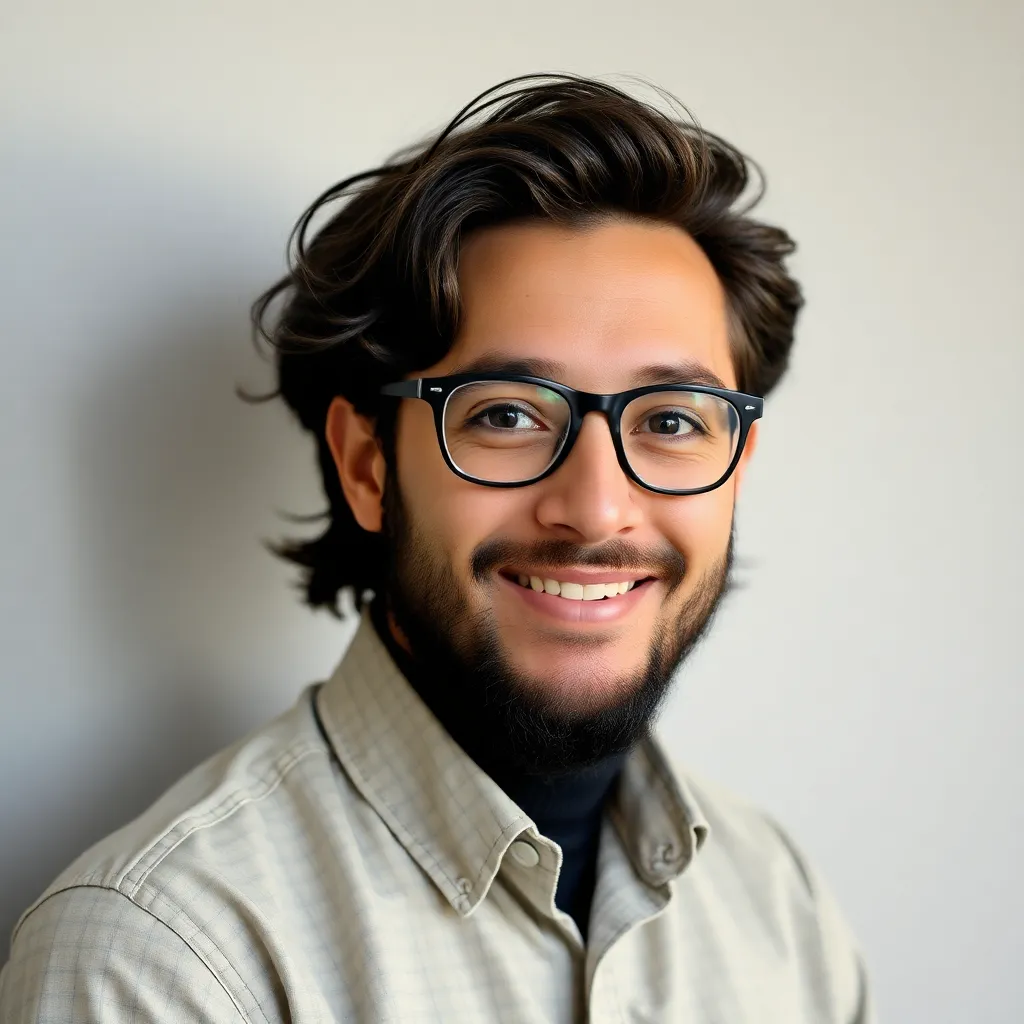
Ronan Farrow
Feb 26, 2025 · 3 min read

Table of Contents
Here's a blog post about downloading YouTube videos using Python. Remember that downloading copyrighted videos without permission is illegal. This information is for educational purposes only.
How to Download YouTube Videos Using Python: A Comprehensive Guide
Downloading YouTube videos can be useful for offline viewing or archival purposes. However, it's crucial to respect copyright laws and only download videos you have permission to access. This guide will walk you through the process using Python, focusing on ethical and legal considerations.
Understanding the Legal Landscape
Before diving into the code, let's address the elephant in the room: copyright. Downloading copyrighted videos without the owner's permission is illegal in most jurisdictions. Always ensure you have the right to download any video before proceeding. This guide is for educational purposes only and should not be used to infringe on copyright.
Choosing the Right Library: pytube
Several Python libraries can download YouTube videos, but pytube
stands out for its ease of use and robust features. It's specifically designed for this purpose and simplifies the process significantly.
Installing pytube
You can install pytube
using pip, Python's package installer:
pip install pytube
Downloading a YouTube Video: A Step-by-Step Guide
This section provides a comprehensive guide on how to download YouTube videos using pytube
. We will cover different aspects such as selecting resolutions, downloading audio only, and handling potential errors.
Step 1: Importing the Library
First, import the necessary library:
from pytube import YouTube
Step 2: Creating a YouTube
Object
Next, create a YouTube
object by providing the video URL:
yt = YouTube("YOUR_YOUTUBE_VIDEO_URL") # Replace with the actual URL
Remember to replace "YOUR_YOUTUBE_VIDEO_URL"
with the actual URL of the YouTube video you want to download.
Step 3: Selecting the Stream
YouTube videos are available in various resolutions and formats. You can use streams.filter()
to select the desired stream based on resolution, file type (e.g., mp4, webm), and other parameters:
stream = yt.streams.filter(progressive=True, file_extension='mp4').first() #Download the first available progressive mp4
#OR
# stream = yt.streams.get_highest_resolution() # Download the highest resolution
#OR
# stream = yt.streams.get_audio_only() # Download only the audio
progressive=True
ensures you get a video stream with both audio and video combined into a single file. Adjust the parameters to fit your needs.
Step 4: Downloading the Video
Finally, download the selected stream:
stream.download(output_path="./downloads") #Download the video to the specified folder
This will download the video to a folder named "downloads" in your current working directory. You can change the output_path
argument to specify a different location.
Handling Errors
It's always a good practice to include error handling in your code. You can use try...except
blocks to catch potential errors, such as network issues or invalid URLs:
try:
yt = YouTube("YOUR_YOUTUBE_VIDEO_URL")
stream = yt.streams.filter(progressive=True, file_extension='mp4').first()
stream.download(output_path="./downloads")
print("Download complete!")
except Exception as e:
print(f"An error occurred: {e}")
Advanced Techniques and Considerations
- Progress Bar: You can enhance the user experience by adding a progress bar to show the download progress. Libraries like
tqdm
can help achieve this. - Multiple Downloads: You can adapt the code to download multiple videos in a loop by reading video URLs from a file or list.
- Authentication: Some videos might require authentication.
pytube
doesn't directly handle this; you may need additional libraries or techniques if you encounter such scenarios.
This comprehensive guide helps you download YouTube videos using Python and pytube
. Remember that respecting copyright laws is paramount. Use this knowledge responsibly and ethically.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How To Delete Linkedin Update | Feb 26, 2025 |
How To Know Your Native American Spirit Animal | Feb 26, 2025 |
How To Delete Apps In Apple Settings | Feb 26, 2025 |
How To Lose A Guy In 10 Days Tony | Feb 26, 2025 |
How To Introduce Yourself Using Adjectives | Feb 26, 2025 |
Latest Posts
Thank you for visiting our website which covers about How To Download Video From Youtube Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.